using System;
using System.Collections;
using System.Collections.Generic;
using System.Diagnostics;
using UnityEngine;
using Debug = UnityEngine.Debug;
public class ResTest2 : MonoBehaviour
{
private AssetBundleManifest abm;
private List<string> absList = new List<string>();
private List<AssetBundle> abs = new List<AssetBundle>();
// Use this for initialization
void Start()
{
StartCoroutine(LoadABM(ABMLoaded));
}
IEnumerator LoadABM(Action action)
{
WWW www = new WWW(Application.streamingAssetsPath + "/windows.t");
yield return www;
if (string.IsNullOrEmpty(www.error))
{
abm = www.assetBundle.LoadAsset<AssetBundleManifest>("AssetBundleManifest");
}
www.Dispose();
www = null;
if (action != null)
{
action();
}
}
void ABMLoaded()
{
Debug.Log("ABM加载完成");
LoadAllAB();
}
#region 测试整体的预加载耗时
Stopwatch load = new Stopwatch();
#endregion
/// <summary>
/// 没有依赖文件的AB
/// </summary>
Dictionary<string, AB> lABs = new Dictionary<string, AB>();
/// <summary>
/// 有依赖文件的AB
/// </summary>
Dictionary<string, AB> dABs = new Dictionary<string, AB>();
/// <summary>
/// 加载所有AB文件
/// </summary>
private void LoadAllAB()
{
#region 测试整体的预加载耗时
load.Start();
#endregion
string[] abNames = abm.GetAllAssetBundles();
Debug.LogWarning("AB总数:" + abNames.Length);
foreach (string abName in abNames)
{
string[] dNames = abm.GetAllDependencies(abName);
if (dNames != null && dNames.Length > 0)
{
AB ab = new AB();
ab.name = abName;
foreach (string dName in dNames)
{
AB dAB = new AB();
dAB.name = dName;
ab.dABs.Add(dAB);
}
if (!dABs.ContainsKey(abName))
{
dABs.Add(abName, ab);
}
}
else
{
AB ab = new AB();
ab.name = abName;
if (!lABs.ContainsKey(abName))
{
lABs.Add(abName, ab);
}
}
}
Debug.LogWarning("无依赖:" + lABs.Count);
Debug.LogWarning("有依赖:" + dABs.Count);
labCount = lABs.Count;
foreach (AB ab in lABs.Values)
{
StartCoroutine(LoadLAB(ab, OnLLoaded));
}
}
private void OnLLoaded()
{
Debug.LogWarning("无依赖的AB加载完毕");
labCount = dABs.Count;
foreach (AB ab in dABs.Values)
{
StartCoroutine(LoadLAB(ab, OnDLoaded));
}
}
private void OnDLoaded()
{
#region 测试预加载整体耗时
load.Stop();
Debug.LogWarning("加载耗时:" + load.ElapsedMilliseconds);
load.Reset();
#endregion
Debug.LogWarning("有依赖的AB加载完毕");
LoadABI("lobby.ui.lobbyroot", null);
}
/// <summary>
/// 无依赖的ab数
/// </summary>
private int labCount = 0;
/// <summary>
/// 已加载的无依赖ab数
/// </summary>
private int lloadedCount = 0;
/// <summary>
/// 加载无依赖的AB
/// </summary>
/// <param name="abName"></param>
/// <param name="action"></param>
/// <returns></returns>
private IEnumerator LoadLAB(AB ab, Action action)
{
WWW www = new WWW(Application.streamingAssetsPath + "/" + ab.name + ".t");
yield return www;
if (string.IsNullOrEmpty(www.error))
{
AssetBundle assetBundle = www.assetBundle;
ab.ab = assetBundle;
ab.isLoaded = true;
www.Dispose();
www = null;
lloadedCount += 1;
if (lloadedCount == labCount)
{
labCount = 0;
lloadedCount = 0;
if (action != null)
{
action();
}
}
}
else
{
Debug.LogWarning("www.error:" + www.error);
}
}
private void LoadABI(string abName, Action action)
{
#region 测试整体的预加载耗时
load.Start();
#endregion
AB ab = null;
dABs.TryGetValue(abName, out ab);
if (ab != null)
{
UnityEngine.Object[] assets = ab.ab.LoadAllAssets();
GameObject go = Instantiate(assets[0]) as GameObject;
}
#region 测试预加载整体耗时
load.Stop();
Debug.LogWarning("加载耗时:" + load.ElapsedMilliseconds);
load.Reset();
#endregion
//WWW www = new WWW(Application.streamingAssetsPath + "/" + abName + ".t");
//yield return www;
//if (string.IsNullOrEmpty(www.error))
//{
// AssetBundle ab = www.assetBundle;
// abs.Add(ab);
// UnityEngine.Object[] assets = ab.LoadAllAssets();
// www.Dispose();
// www = null;
// absList.Add(abName);
// GameObject go = Instantiate(assets[0]) as GameObject;
// yield return new WaitForEndOfFrame();
//}
}
private void OnApplicationQuit()
{
for (int i = 0; i < abs.Count; i++)
{
abs[i].Unload(true);
abs[i] = null;
}
abs.Clear();
absList.Clear();
Resources.UnloadUnusedAssets();
}
}
using System.Collections.Generic;
using UnityEngine;
/// <summary>
/// AssetBundle Item
/// </summary>
public class AB
{
/// <summary>
/// abname
/// </summary>
public string name = "";
public AssetBundle ab = null;
/// <summary>
/// 依赖文件
/// </summary>
public List<AB> dABs = new List<AB>();
/// <summary>
/// 是否已加载
/// </summary>
public bool isLoaded = false;
}
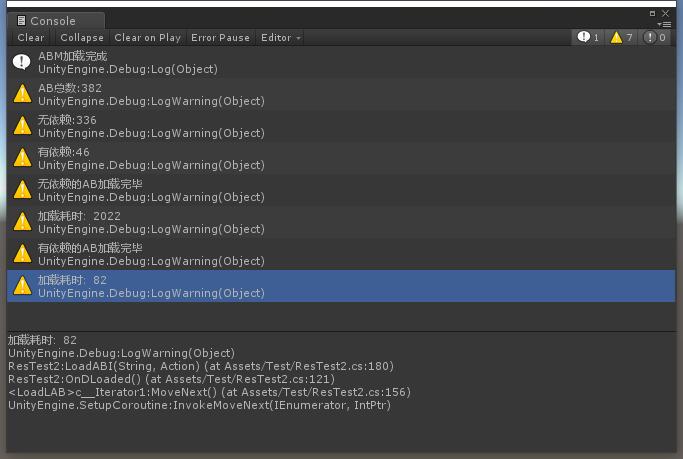
Good