1、安卓端代码
package cn.net.xuefei.schemedemo;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import com.unity3d.player.UnityPlayer;
import com.unity3d.player.UnityPlayerActivity;
public class MainActivity extends UnityPlayerActivity {
private String launchInfo="";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
launchInfo = onLaunchInfo();
}
@Override
public void onResume()
{
super.onResume();
launchInfo = onLaunchInfo();
}
private String onLaunchInfo()
{
String info="";
Uri uri = getIntent().getData();
if (uri != null) {
// 完整的url信息
String url = uri.toString();
info = url;
Log.e("Unity", "url: " + url);
}
return info;
}
public void getLaunchInfo()
{
UnityPlayer.UnitySendMessage("Main Camera", "OnLaunchInfo", launchInfo);
launchInfo="";
}
}
2、Unity中 AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package = "cn.net.xuefei.schemedemo"
android:versionCode="1"
android:versionName="1.0">
<application
android:allowBackup="true"
android:icon="@drawable/app_icon"
android:label="@string/app_name"
android:supportsRtl="true">
<activity android:name=".MainActivity" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.BROWSABLE" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="scheme"
android:host="host"
android:path="/path"
android:port="8888"/>
</intent-filter>
<meta-data android:name="unityplayer.UnityActivity" android:value="true" />
</activity>
</application>
</manifest>
3、Unity中代码
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
#if UNITY_IOS
using System.Runtime.InteropServices;
#endif
public class SchemeDemo : MonoBehaviour
{
public Text text;
// Use this for initialization
void Start()
{
GetInfo();
}
// Update is called once per frame
void Update()
{
}
public void OnLaunchInfo(string launchInfo)
{
Debug.LogError("launchInfo:" + launchInfo);
text.text = launchInfo;
}
public void GetInfo()
{
#if UNITY_ANDROID
AndroidJavaClass jc = new AndroidJavaClass("com.unity3d.player.UnityPlayer");
AndroidJavaObject jo = jc.GetStatic<AndroidJavaObject>("currentActivity");
jo.Call("getLaunchInfo");
#elif UNITY_IOS
_GetLaunchInfo();
#endif
}
private void OnApplicationFocus(bool focus)
{
Debug.LogError("focus:" + focus);
if (true)
{
GetInfo();
}
}
#if UNITY_IOS
[DllImport("__Internal")]
private static extern void _GetLaunchInfo();
#endif
}
4、设置Unity iOS URL Schemes,修改导出的Xcode工程中的UnityAppController.mm
#import "UnityAppController.h"
NSString *URLString = @"";
// 向Unity传递参数;
extern void UnitySendMessage(const char *, const char *, const char *);
//添加的代码
- (BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<NSString *,id> *)options
{
URLString = [url absoluteString];
return YES;
}
- (BOOL)application:(UIApplication*)application openURL:(NSURL*)url sourceApplication:(NSString*)sourceApplication annotation:(id)annotation
{
//添加的代码
URLString = [url absoluteString];
return YES;
}
extern "C"
{
void _GetLaunchInfo();
}
void _GetLaunchInfo()
{
UnitySendMessage( "Main Camera", [@"OnLaunchInfo" UTF8String], [URLString UTF8String] );
// 清空,防止造成干扰;
URLString = @"";
}
5、网页
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input id="btn" type="button" value="唤起SchemeDemo">
<script>
var btn = document.getElementById('btn');
btn.onclick = function () {
jump('scheme://host:8888/path?ps=123456');
};
function GetMobelType() {
var browser = {
versions: function() {
var u = window.navigator.userAgent;
console.log(u); //Safari浏览器 Mozilla/5.0 (Macintosh; Intel Mac OS X 10_13_5) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/11.1.1 Safari/605.1.15
return {
trident: u.indexOf('Trident') > -1, //IE内核
presto: u.indexOf('Presto') > -1, //opera内核
Alipay: u.indexOf('Alipay') > -1, //支付宝
webKit: u.indexOf('AppleWebKit') > -1, //苹果、谷歌内核
gecko: u.indexOf('Gecko') > -1 && u.indexOf('KHTML') == -1, //火狐内核
mobile: !!u.match(/AppleWebKit.*Mobile.*/), //是否为移动终端
ios: !!u.match(/\(i[^;]+;( U;)? CPU.+Mac OS X/), //ios终端
android: u.indexOf('Android') > -1 || u.indexOf('Linux') > -1, //android终端或者uc浏览器
iPhone: u.indexOf('iPhone') > -1 || u.indexOf('Mac') > -1, //是否为iPhone或者安卓QQ浏览器
//iPhone: u.match(/iphone|ipod|ipad/),//
iPad: u.indexOf('iPad') > -1, //是否为iPad
webApp: u.indexOf('Safari') == -1, //是否为web应用程序,没有头部与底部
weixin: u.indexOf('MicroMessenger') > -1, //是否为微信浏览器
qq: u.match(/\sQQ/i) !== null, //是否QQ
Safari: u.indexOf('Safari') > -1,
///Safari浏览器,
};
}()
};
return browser.versions;
}
function jump(myurl) {
var timeout = 2300, timer = null;
if(GetMobelType().weixin) {
// 微信浏览器不支持跳转
// 可以显示提示在其他浏览器打开
} else {
var startTime = Date.now();
if(GetMobelType().android) {
var ifr = document.createElement('iframe');
ifr.src = myurl;//这里是唤起App的协议,有Android可爱的同事提供
ifr.style.display = 'none';
document.body.appendChild(ifr);
timer = setTimeout(function() {
var endTime = Date.now();
if(!startTime || endTime - startTime < timeout + 300) {
document.body.removeChild(ifr);
//window.open("唤起失败跳转的链接");
window.open("https://www.ganghood.net.cn/SchemeDemo.apk");
}
}, timeout);
}
if(GetMobelType().ios || GetMobelType().iPhone || GetMobelType().iPad) {
if(GetMobelType.qq) {
// ios的苹果浏览器
// 提示在浏览器打开的蒙板
} else {
/*var ifr = document.createElement("iframe");
ifr.src = myurl;
ifr.style.display = "none";*/ // iOS9+不支持iframe唤起app
window.location.href = myurl; //唤起协议,由iOS小哥哥提供
//document.body.appendChild(ifr);
timer = setTimeout(function() {
// window.location.href = "ios下载的链接";
window.location.href = "https://www.ganghood.net.cn/没有该文件.ipa";
}, timeout);
};
}
}
}
</script>
</body>
</html>
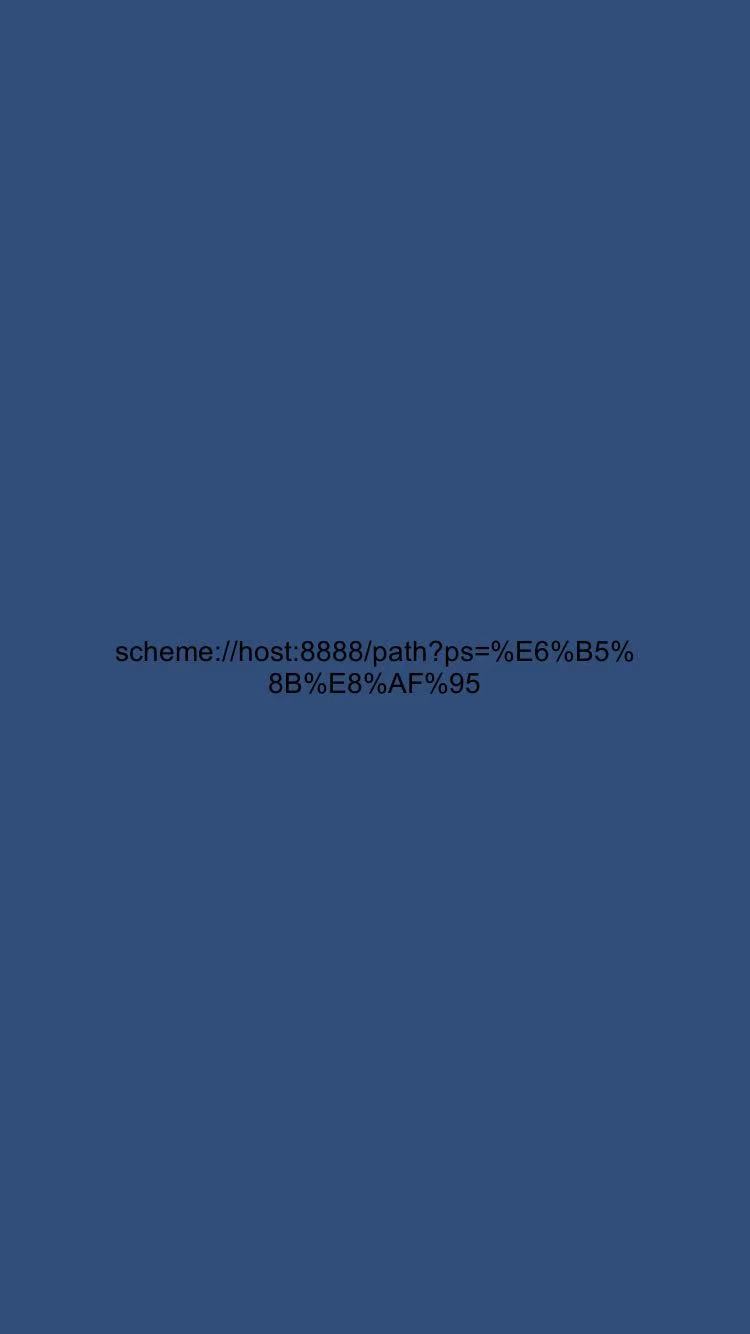
工程地址https://gitee.com/awnuxcvbn/SchemeDemo